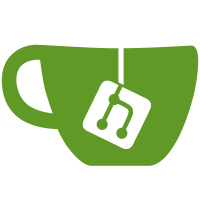
When running UI tests locally, we found that the `android-device-provider-local` dependency is no longer available on mavenCentral. Our dependency repository uses various regex to allow only google dependencies to be fetched from Google Maven, but somehow these did not include the test ones everywhere.
249 lines
8.1 KiB
Groovy
249 lines
8.1 KiB
Groovy
// Top-level build file where you can add configuration options common to all sub-projects/modules.
|
|
|
|
import org.mozilla.fenix.gradle.tasks.GithubDetailsTask
|
|
|
|
buildscript {
|
|
// This logic is duplicated in the allprojects block: I don't know how to fix that.
|
|
repositories {
|
|
maven {
|
|
name "Mozilla Nightly"
|
|
url "https://nightly.maven.mozilla.org/maven2"
|
|
content {
|
|
// Improve performance: only check moz maven for mozilla deps.
|
|
includeGroupByRegex RepoMatching.mozilla
|
|
}
|
|
}
|
|
|
|
maven {
|
|
name "Mozilla"
|
|
url "https://maven.mozilla.org/maven2"
|
|
content {
|
|
// Improve performance: only check moz maven for mozilla deps.
|
|
includeGroupByRegex RepoMatching.mozilla
|
|
}
|
|
}
|
|
|
|
if (project.hasProperty("googleRepo")) {
|
|
maven {
|
|
name "Google"
|
|
allowInsecureProtocol true // Local Nexus in CI uses HTTP
|
|
url project.property("googleRepo")
|
|
}
|
|
} else {
|
|
google() {
|
|
content {
|
|
// Improve performance: only check google maven for google deps.
|
|
includeGroupByRegex RepoMatching.androidx
|
|
includeGroupByRegex RepoMatching.comGoogleAndroid
|
|
includeGroupByRegex RepoMatching.comGoogleFirebase
|
|
includeGroupByRegex RepoMatching.comGoogleTesting
|
|
includeGroupByRegex RepoMatching.comAndroid
|
|
}
|
|
}
|
|
}
|
|
|
|
if (project.hasProperty("centralRepo")) {
|
|
maven {
|
|
name "MavenCentral"
|
|
url project.property("centralRepo")
|
|
allowInsecureProtocol true // Local Nexus in CI uses HTTP
|
|
}
|
|
} else {
|
|
mavenCentral() {
|
|
content {
|
|
// Improve security: don't search deps with known repos.
|
|
excludeGroupByRegex RepoMatching.mozilla
|
|
excludeGroupByRegex RepoMatching.androidx
|
|
excludeGroupByRegex RepoMatching.comGoogleAndroid
|
|
excludeGroupByRegex RepoMatching.comGoogleFirebase
|
|
excludeGroupByRegex RepoMatching.comGoogleTesting
|
|
excludeGroupByRegex RepoMatching.comAndroid
|
|
}
|
|
}
|
|
}
|
|
}
|
|
|
|
dependencies {
|
|
classpath Deps.tools_androidgradle
|
|
classpath Deps.tools_kotlingradle
|
|
classpath Deps.tools_benchmarkgradle
|
|
classpath Deps.androidx_safeargs
|
|
classpath Deps.osslicenses_plugin
|
|
|
|
classpath "org.mozilla.components:tooling-glean-gradle:${Versions.mozilla_android_components}"
|
|
classpath "org.mozilla.components:tooling-nimbus-gradle:${Versions.mozilla_android_components}"
|
|
|
|
// NOTE: Do not place your application dependencies here; they belong
|
|
// in the individual module build.gradle files
|
|
}
|
|
}
|
|
|
|
plugins {
|
|
id("io.gitlab.arturbosch.detekt").version("1.19.0")
|
|
}
|
|
|
|
allprojects {
|
|
// This logic is duplicated in the buildscript block: I don't know how to fix that.
|
|
repositories {
|
|
maven {
|
|
name "Mozilla Nightly"
|
|
url "https://nightly.maven.mozilla.org/maven2"
|
|
content {
|
|
// Improve performance: only check moz maven for mozilla deps.
|
|
includeGroupByRegex RepoMatching.mozilla
|
|
}
|
|
}
|
|
|
|
maven {
|
|
name "Mozilla"
|
|
url "https://maven.mozilla.org/maven2"
|
|
content {
|
|
// Improve performance: only check moz maven for mozilla deps.
|
|
includeGroupByRegex RepoMatching.mozilla
|
|
}
|
|
}
|
|
|
|
if (project.hasProperty("googleRepo")) {
|
|
maven {
|
|
name "Google"
|
|
url project.property("googleRepo")
|
|
allowInsecureProtocol true // Local Nexus in CI uses HTTP
|
|
}
|
|
} else {
|
|
google() {
|
|
content {
|
|
// Improve performance: only check google maven for google deps.
|
|
includeGroupByRegex RepoMatching.androidx
|
|
includeGroupByRegex RepoMatching.comGoogleAndroid
|
|
includeGroupByRegex RepoMatching.comGoogleFirebase
|
|
includeGroupByRegex RepoMatching.comGoogleTesting
|
|
includeGroupByRegex RepoMatching.comAndroid
|
|
}
|
|
}
|
|
}
|
|
|
|
if (project.hasProperty("centralRepo")) {
|
|
maven {
|
|
name "MavenCentral"
|
|
url project.property("centralRepo")
|
|
allowInsecureProtocol true // Local Nexus in CI uses HTTP
|
|
}
|
|
} else {
|
|
mavenCentral() {
|
|
content {
|
|
// Improve security: don't search deps with known repos.
|
|
excludeGroupByRegex RepoMatching.mozilla
|
|
excludeGroupByRegex RepoMatching.androidx
|
|
excludeGroupByRegex RepoMatching.comGoogleAndroid
|
|
excludeGroupByRegex RepoMatching.comGoogleFirebase
|
|
excludeGroupByRegex RepoMatching.comGoogleTesting
|
|
excludeGroupByRegex RepoMatching.comAndroid
|
|
}
|
|
}
|
|
}
|
|
}
|
|
|
|
tasks.withType(org.jetbrains.kotlin.gradle.tasks.KotlinCompile).configureEach {
|
|
kotlinOptions.jvmTarget = "1.8"
|
|
kotlinOptions.allWarningsAsErrors = true
|
|
kotlinOptions.freeCompilerArgs += [
|
|
"-opt-in=kotlin.RequiresOptIn", "-Xjvm-default=all"
|
|
]
|
|
}
|
|
}
|
|
|
|
tasks.register('clean', Delete) {
|
|
delete rootProject.buildDir
|
|
}
|
|
|
|
detekt {
|
|
input = files("$projectDir/app/src")
|
|
config = files("$projectDir/config/detekt.yml")
|
|
|
|
reports {
|
|
html {
|
|
enabled = true
|
|
destination = file("$projectDir/build/reports/detekt.html")
|
|
}
|
|
xml {
|
|
enabled = false
|
|
}
|
|
txt {
|
|
enabled = false
|
|
}
|
|
}
|
|
}
|
|
|
|
tasks.withType(io.gitlab.arturbosch.detekt.Detekt).configureEach() {
|
|
autoCorrect = true
|
|
|
|
exclude "**/test/**"
|
|
exclude "**/androidTest/**"
|
|
exclude "**/build/**"
|
|
exclude "**/resources/**"
|
|
exclude "**/tmp/**"
|
|
}
|
|
|
|
// Apply same path exclusions as for the main task
|
|
tasks.withType(io.gitlab.arturbosch.detekt.DetektCreateBaselineTask).configureEach() {
|
|
exclude "**/test/**"
|
|
exclude "**/androidTest/**"
|
|
exclude "**/build/**"
|
|
exclude "**/resources/**"
|
|
exclude "**/tmp/**"
|
|
}
|
|
|
|
configurations {
|
|
ktlint
|
|
}
|
|
|
|
dependencies {
|
|
ktlint("com.pinterest:ktlint:0.47.0") {
|
|
attributes {
|
|
attribute(Bundling.BUNDLING_ATTRIBUTE, getObjects().named(Bundling, Bundling.EXTERNAL))
|
|
}
|
|
}
|
|
|
|
detekt project(":mozilla-detekt-rules")
|
|
detekt "io.gitlab.arturbosch.detekt:detekt-cli:${Versions.detekt}"
|
|
}
|
|
|
|
tasks.register('ktlint', JavaExec) {
|
|
group = "verification"
|
|
description = "Check Kotlin code style."
|
|
classpath = configurations.ktlint
|
|
main = "com.pinterest.ktlint.Main"
|
|
args "app/src/**/*.kt", "!**/build/**/*.kt", "--baseline=ktlint-baseline.xml"
|
|
}
|
|
|
|
task ktlintFormat(type: JavaExec, group: "formatting") {
|
|
description = "Fix Kotlin code style deviations."
|
|
classpath = configurations.ktlint
|
|
main = "com.pinterest.ktlint.Main"
|
|
args "-F", "app/src/**/*.kt", "!**/build/**/*.kt", "--baseline=ktlint-baseline.xml"
|
|
}
|
|
|
|
tasks.withType(io.gitlab.arturbosch.detekt.Detekt.class).configureEach {
|
|
exclude("**/resources/**")
|
|
exclude("**/tmp/**")
|
|
}
|
|
|
|
tasks.register("listRepositories") {
|
|
doLast {
|
|
println "Repositories:"
|
|
project.repositories.each { println "Name: " + it.name + "; url: " + it.url }
|
|
}
|
|
}
|
|
|
|
tasks.register("githubTestDetails", GithubDetailsTask) {
|
|
text = "### [Unit Test Results]({reportsUrl}/test/testDebugUnitTest/index.html)"
|
|
}
|
|
|
|
tasks.register("githubLintDetektDetails", GithubDetailsTask) {
|
|
text = "### [Detekt Results]({reportsUrl}/detekt.html)"
|
|
}
|
|
|
|
tasks.register("githubLintAndroidDetails", GithubDetailsTask) {
|
|
text = "### [Android Lint Results]({reportsUrl}/lint-results-debug.html)"
|
|
}
|